Batch Image Processing Your First Batch Request
Don't have a Batch Service yet? Click Here!
There are a few requirements in order to make your first batch request, these are:
- Service ID: This can be found inside your service page on the SlashedCloud dashboard.
- Service Secret Key: This can be found inside your service page on the SlashedCloud dashboard.
- API Endpoint:
https://batch.slashed.cloud
- Source: The path of your image source.
- Destination: The path of your image destination.
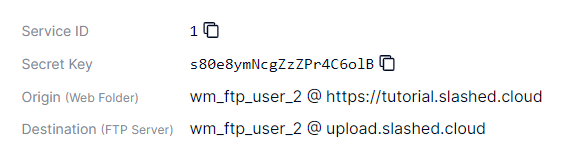
Example of what you see in your service page.
What does a batch request look like?
Parameters
service_id
: number Your service ID, which is a unique identifier for the service you are using. It's essential to include this in your request so that the server knows which service you are accessing.
callback
: string This is the callback URL. After the server processes your batch request and completes the specified transformations, it will send the response back to this URL.
source
: string The source file that you want to transform. The source file must be in the storage that you have set up.
jobs
: array This is an array of jobs to be applied to the source file - In this example we have a transformation.
{
"service_id": 1,
"source": "/480x360/1.jpg",
"jobs": [
{
"destination": "/480x360/transformed/1.jpg",
"quality": 70,
"transformations": [
{
"type": "annotation",
"font": "Arial",
"color": "#000000",
"text": "Test text",
"size": 10,
"gravity": "center"
}
]
}
]
}
1.1 Jobs
Each job has its own destination, quality parameter and transformation array. Below are the parameters for each.
1.2.1 Batch Job Parameters
destination
: string The destination of the transformed file. The destination file must be in the storage that you have set up.
quality
: string The quality of the transformed file. The quality can be a number between 0 and 100. Default is 70.
transformations
: array This is an array of transformations to be applied to the source file.
[
{
"destination": "/480x360/transformed/1.jpg",
"quality": 70,
"transformations": [
{
"type": "annotation",
"font": "Arial",
"color": "#000000",
"text": "Test text",
"size": 10,
"gravity": "center"
}
]
}
]
1.2 Transformations
1.2.1 Annotation
type
: 'annotation'
font
: string This parameter sets the font. Must be a string.
color
: string This parameter sets the color. Must be hexadecimal.
text
: string This parameter sets the text. Must be a string.
size
: number This parameter sets the size. Must be a number.
gravity
: string This parameter sets the gravity. Must be one of 'center', 'north', 'northeast', 'east', 'southeast', 'south', 'southwest', 'west', 'northwest'.
xoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
yoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
{
"type": "annotation",
"font": "Arial",
"color": "#000000",
"text": "Test text",
"size": 10,
"gravity": "center"
}
1.2.2 Blur
type
: 'blur'
sigma
: number This parameter sets the sigma. Must be a number.
radius
: number This parameter sets the radius. Must be a number.
{
"type": "blur",
"sigma": 218,
"radius": 465
}
1.2.3 Brightness
type
: 'brightness'
bright_level
: number This parameter sets the brightness level. Must be a number between -255 and 255.
{
"type": "brightness",
"bright_level": 230
}
1.2.4 Colorize
type
: 'colorize'
red
: number This parameter sets the red level. Must be a number between 0 and 255.
green
: number This parameter sets the green level. Must be a number between 0 and 255.
blue
: number This parameter sets the blue level. Must be a number between 0 and 255.
{
"type": "colorize",
"red": 200,
"green": 120,
"blue": 150
}
1.2.5 Contrast
type
: 'contrast'
level
: number This parameter sets the contrast level. Must be a number between -100 and 100.
{
"type": "contrast",
"level": 55
}
1.2.6 Crop
type
: 'crop'
gravity
: string This parameter sets the gravity. Must be one of 'center', 'north', 'northeast', 'east', 'southeast', 'south', 'southwest', 'west', 'northwest'.
width
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original width: "100%"
Percentage of the original height: "100h"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
height
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original height: "100%"
Percentage of the original width: "100w"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
xoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
yoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
fit
: boolean This parameter calculates the best fit. Must be a boolean. Defaults to true.
Ensures that the image fits within certain dimensions (presumably maximum dimensions)
while maintaining its original aspect ratio, if the fit parameter is set to true. It's a
way to prevent the image from becoming too large or too distorted during resizing.
{
"type": "crop",
"gravity": "center",
"width": "750",
"height": "500",
"yoffset": "300",
"xoffset": "500",
"fit": true
}
1.2.7 Enhance
type
: 'enhance'
{
"type": "enhance"
}
1.2.8 Filter
type
: 'filter'
filter
: string This parameter sets the filter. Must be one of 'bw', 'sepia', 'negate', 'grayscale', 'edgedetect', 'emboss', 'blurgaussian', 'blurselective', 'meanremoval', 'smooth'.
{
"type": "filter",
"filter": "sepia"
}
1.2.9 Fitmethod
type
: 'fitmethod'
color
: string This parameter sets the color. Must be hexadecimal.
fit_type
: string This parameter sets the fit type. Must be one of 'box', 'stretch', 'cropbox', 'letterbox', 'outside'.
gravity
: string This parameter sets the gravity. Must be one of 'center', 'north', 'northeast', 'east', 'southeast', 'south', 'southwest', 'west', 'northwest'.
width
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original width: "100%"
Percentage of the original height: "100h"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
height
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original height: "100%"
Percentage of the original width: "100w"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
opacity
: number This parameter sets the opacity. Must be a number between 0 and 10.
{
"type": "fitmethod",
"color": "#000000",
"fit_type": "cropbox",
"gravity": "center",
"width": 550,
"height": 300,
"opacity": 0
}
1.2.10 Flip
type
: 'flip'
direction
: string This parameter sets the direction of the flip. Must be one of 'x', 'y', 'vertical' or 'horizontal'.
{
"type": "flip",
"direction": "y"
}
1.2.11 Resize
type
: 'resize'
width
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original width: "100%"
Percentage of the original height: "100h"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
height
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original height: "100%"
Percentage of the original width: "100w"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
{
"type": "resize",
"width": 500,
"height": 300
}
1.2.12 Rotate
type
: 'rotate'
degrees
: number This parameter sets the degrees of the rotation. Must be a number between 0 and 360 with up to two decimal places.
{
"type": "rotate",
"degrees": 20
}
1.2.13 Ulb
type
: 'ulb'
{
"type": "ulb"
}
1.2.14 Watermark
type
: 'watermark'
gravity
: string This parameter sets the gravity. Must be one of 'center', 'north', 'northeast', 'east', 'southeast', 'south', 'southwest', 'west', 'northwest'.
width
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original width: "100%"
Percentage of the original height: "100h"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
height
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original height: "100%"
Percentage of the original width: "100w"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
Source
: string This parameter sets the source of the watermark.
xoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
yoffset
: string | number Can be a negative value. Possible values:
Pixels: 100
, "100"
or "100px"
Percentage: "100%"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
{
"type": "watermark",
"gravity": "middle",
"width": 200,
"height": 200,
"source": "watermark.png",
"yoffset": 0,
"xoffset": 0
}
1.2.15 Zoom
type
: 'zoom'
width
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original width: "100%"
Percentage of the original height: "100h"
Width Parameter with Percentage (%): Only one of 'width' or 'height' is required.
height
: string | number Possible values:
Pixels: 100
, "100"
or "100px"
Percentage of the original height: "100%"
Percentage of the original width: "100w"
Height Parameter with Percentage (%): Only one of 'width' or 'height' is required.
level
: number This parameter sets the level of zoom. Must be a number from 0 to 10.
{
"type": "zoom",
"width": "200",
"height": "200",
"level": 0
}
How to send a Batch request?
Head over to Postman:
- Method: Set the Method to
POST
. - API URL: Set the URL to
https://batch.slashed.cloud
- Go to Body and select
raw
andJSON
. - Copy and paste the JSON request above into the body.
Note: Please make sure you insert YOUR service ID, source and destination. - Go to Headers and add the following:
Authorization: Bearer YourSecretKey
Content-Type: application/json
- Click
Send
and you should get a response back with the status of your request.
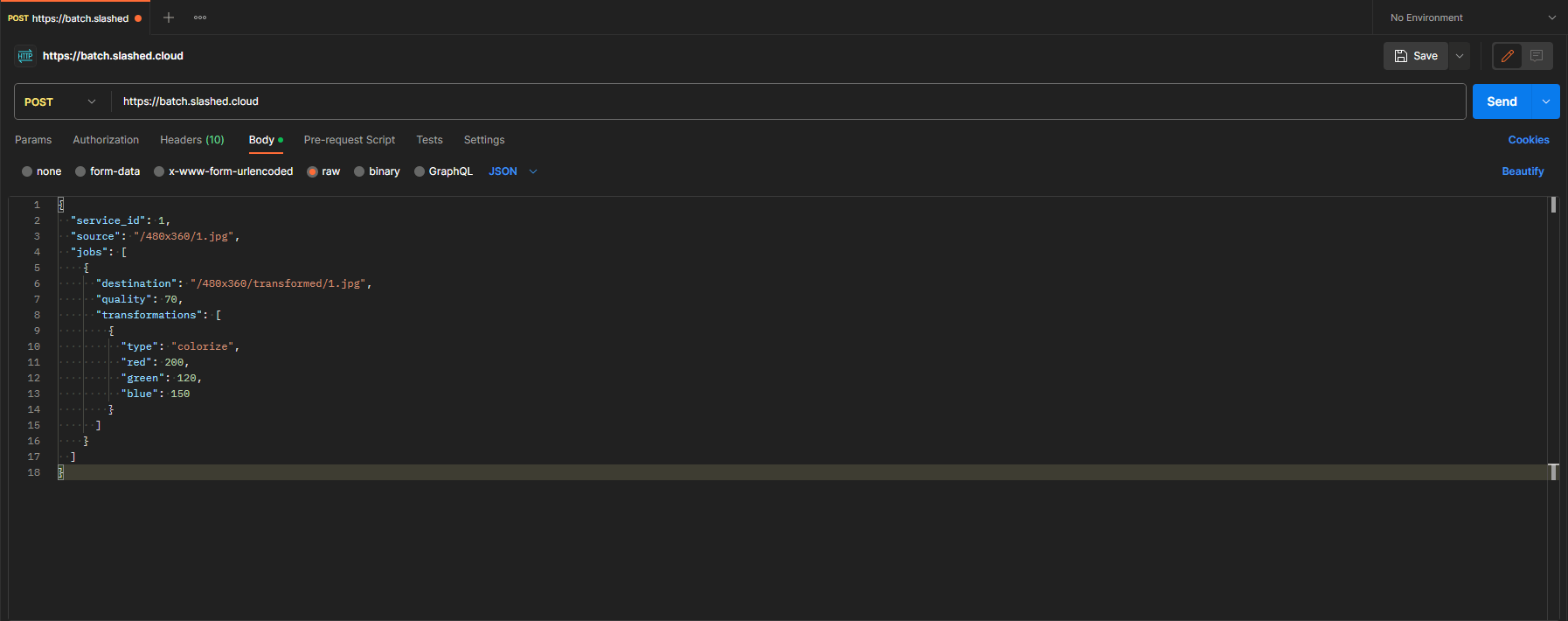
